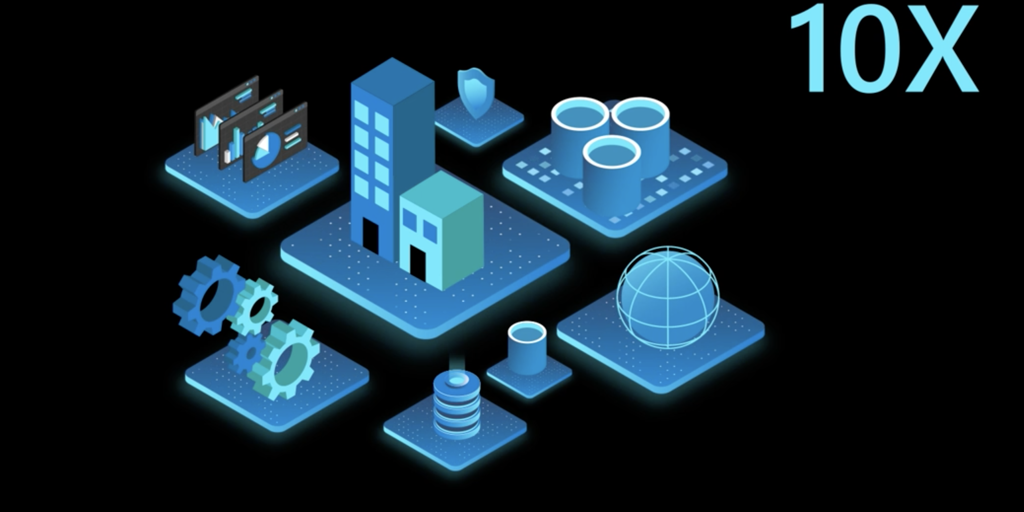
Response caching is a technique used to store the responses of HTTP requests so that subsequent identical requests can be served directly from the cache instead of processing them again. This can significantly improve the performance and scalability of web applications.
In ASP.NET Core, response caching can be implemented using the [ResponseCache]
attribute or by configuring caching options in the application's Startup
class. To use Azure Redis Cache as the caching provider, you need to configure the Redis cache settings in your ASP.NET Core application.
In Umbraco 11 and ASP.NET Core, you can integrate response caching with Azure Redis Cache by following these steps:
Install the required NuGet packages:
Microsoft.Extensions.Caching.StackExchangeRedis
: This package provides the Redis cache functionality.Umbraco.Cms.Core
: This package contains the Umbraco CMS core libraries.
Configure Redis cache in ASP.NET Core: In the
ConfigureServices
method of yourStartup
class, add the Redis cache configuration using theservices.AddStackExchangeRedisCache()
method. Provide the connection string or other necessary details to connect to your Azure Redis Cache instance.using Microsoft.Extensions.Caching.StackExchangeRedis; // ... public void ConfigureServices(IServiceCollection services) { // Other service configurations... services.AddStackExchangeRedisCache(options => { options.Configuration = "your-redis-connection-string"; options.InstanceName = "your-redis-instance-name"; }); // Umbraco CMS services services.AddUmbraco(); }
Replace
your-redis-connection-string
with the connection string of your Azure Redis Cache instance andyour-redis-instance-name
with the desired instance name.Enable response caching in Umbraco: In your
Startup
class, locate theConfigure
method and add the following code to enable response caching:using Microsoft.AspNetCore.Builder; // ... public void Configure(IApplicationBuilder app, IWebHostEnvironment env) { // Other middleware configurations... app.UseResponseCaching(); // Umbraco CMS middleware app.UseUmbraco(); }
Verify caching behavior: With the above configurations in place, Umbraco 11 and ASP.NET Core will use Azure Redis Cache for response caching. Test your Umbraco website by accessing various pages and content. Monitor the performance and ensure that subsequent requests for the same content are served from the cache, reducing the load on the server.
By integrating Azure Redis Cache with Umbraco 11 and ASP.NET Core, you can benefit from distributed caching and improve the performance and scalability of your Umbraco website.
Remember to consider cache invalidation strategies and handle scenarios where the cached data needs to be invalidated or refreshed based on specific events or changes in the application data.
You can invalidate the cache using ContentCacheRefresherNotification
ContentCacheRefresher: INotificationHandler<ContentCacheRefresherNotification>